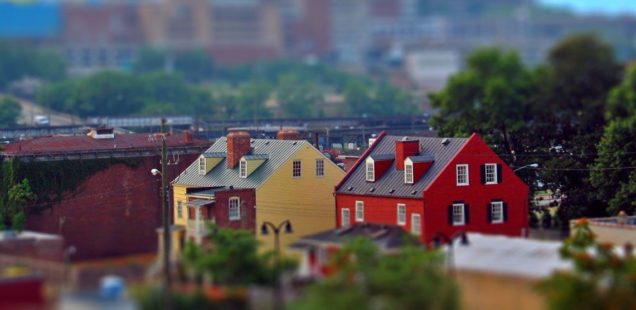
Configuring react-d3 into a Rollup Build System
Rollup is a next generation bundling system that I’m using with react. There is a port of the d3 Javascript graphing library called react-d3. As with most cases where you put together a bunch of new technologies, there are configuration issues.
Here is how I include react-d3 into my rollup projects:
import React from 'react' import { LineChart } from 'react-d3'
Then to make an actual graph:
export class GraphWidget extends React.Component { constructor( props ) { super( props ); } render() { var width = 700, height = 300, margins = {left: 100, right: 100, top: 50, bottom: 50}, title = "Events", x = function( d ) { return d.index; }; var lineData = [ { name: "series1", values: [ { x: 0, y: 20 }, { x: 24, y: 10 } ] }, { name: "series2", values: [ { x: 70, y: 82 }, { x: 76, y: 82 } ] } ]; return ( <LineChart title = {title} width = {width} height = {height} margins = {margins} data = {lineData} x = {x} /> ); } }
React-D3 breaks with Use Strict
The current version of d3
, and react-d3
depends on having access to “this”. The default build with babel is to use strict
, which eliminates the global this, and will break react-d3
.
There is a lot of discussion on the web about ways to turn off use strict
in plain babel environments, and with more popular packagers like webpack and browserify. Here is how I solved this for my rollup environment.
First install:
npm install rollup-plugin-post-replace
This is just like the common plugin rollup-plugin-replace
except that it runs after bundling.
Then in rollup.config.js
:
var postReplaceConfig = { '"use strict";': '', "'use strict';": '' }; var config = { plugins: [ postReplace ( postReplaceConfig ), ] }
Resources
ReactD3 | Main page for the ReactD3 project |
RollupJs | Main page for the RollupJs project |
Babel | Main page for the Babel JS transpiler project |
React | Main page for the React JS framework |
rollup-plugin-post-replace | A rollup plugin to replace text after bundling |
How to remove global “use strict” added by babel | StackOverflow answer about how to do this with plain babel. |