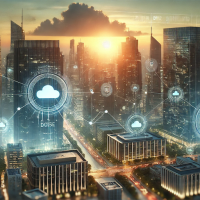
How to build Fastly Compute@ Edge Services in C++
There are a lot of advantages to running code on CDN edge nodes: The latency from the user to your edge service is typically very very low. The services are generally stateless – and so can scale with demand very…
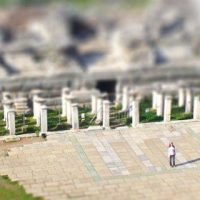
Adding Virtual Tables to Classes after the Fact
Sometimes you want to create classes that are meant to be low level and fast. You don’t want to pay the cost of virtual virtual functions – you want the functions to be inline. But then at other times you…
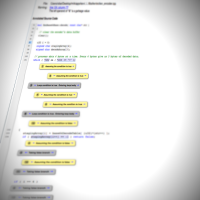
Clang scan-build: a great C++ Static Analyzer
Clang has a command line utility that is very easy to use, and adds a static code analysis step to gcc or clang. It is trivial to install (less than 5 minutes – really! ) and works with almost any…
Using GDB to Dump Program State
Sometimes when a program is running for you want to dump the complete state of all the calls stacks of all threads in a program. This is not so easy to do yourself, but thankfully there are tools like GDB,…
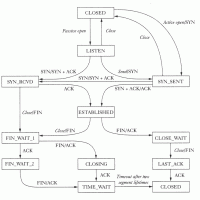
Finding the state of TCP/IP Sockets on Linux
It is not possible through the sockets API to find the connection state of a socket. But on Linux there is a way, a user space way, to find the connection state of a socket. Network information in the Proc…
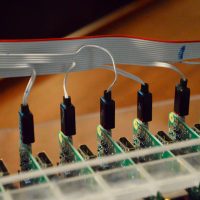
Build GCC with Musl for Raspbian
Musl is a libc replacement designed for embedded systems. It tries to be compatible with POSIX and where possible with glibc, the default c standard library for GCC. Its code is designed to be better for small memory systems. One…
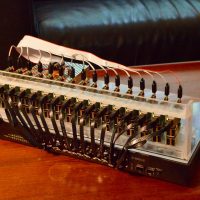
Choosing the Right CPU Flags for GCC on Raspbian
I’ve been messing with the CPU flags for GCC on Raspberry PI. Raspberry PI1 uses a 32 bit ARM6 cpu, the Broadcom BCM2835. The PI2 uses a 32 bit ARM7 cpu, the Broadcom BCM2836. The PI3 uses a 64 bit…
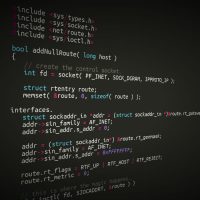
Adding and Removing Routes in the Linux Routing Table
We will go through how to add and remove null routes in c++, though there is a lot more you can do with Linux’ routing tables. For this project I want to use the routing tables like a firewall. I…
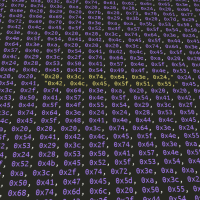
Packing Data Files into Compiled Executables
Have you ever wanted to distribute a compiled binary that included data files packed into the executable file? Embedding a Data File Before Compilation You can do this before compilation by encoding the file into a binary representation, and then…
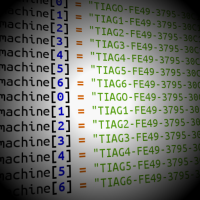
Generating a Cross-Platform Unique Machine Fingerprint
Sometimes you need a program to know if it is running on the same machine. There are a lot of purposes for this, but a common one is if you are writing a licensing module. You want to generate a…